“Onion Ring Bokeh”¶
In the photography community, defects in the out-of-focus highlights of lenses are widely discussed. These result from mid-particular spatial frequency errors on the optical surfaces. Here, we will show an example of using prysm in a relatively extended fashion to model this. We begin, as usual, by importing some classes and other libraries.
[1]:
import numpy as np
from prysm import NollZernike, PSF
from prysm.coordinates import make_rho_phi_grid
from matplotlib import pyplot as plt
%matplotlib inline
We begin by using the NollZernike
class to make a pupil with 25 waves fo defocus to give us a relatively uniform disk for the diffraction limited out of focus image.
[2]:
pupil = NollZernike(Z4=25/1.5, samples=384, dia=25, z_unit='waves') # 100 waves PV of defocus, 25mm for F/2
ps = PSF.from_pupil(pupil, efl=50, Q=1)
ps.plot2d(xlim=200, power=1/3)
[2]:
(<Figure size 432x288 with 2 Axes>,
<AxesSubplot:xlabel='Image Plane X [$\\mathrm{\\mu m}$]', ylabel='Image Plane Y [$\\mathrm{\\mu m}$]'>)
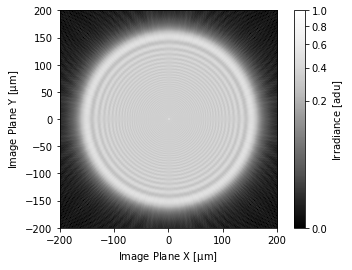
The ripples are Fresnel rings and are a consequence interference very similar to the Gibbs phenomenon. They disappear the farther out of focus you go, and can be wiped away by coarser sampling with an image sensor, or significantly polychromatic light.
We used a value of Q below 2 (worse than Nyquist sampled) because the image is so out of focus, it largely lacks high spatial frequency content to be aliased, so the choice of Q has minimal consequence.
What if the pupil had some ripples in it from structured errors on optical surfaces in the system?
[3]:
pupil2 = pupil.copy()
rho, _ = make_rho_phi_grid(samples_x=pupil.samples_x)
# 15 cycles per aperture, lambda/25 RMS amplitude
const = 2 * np.pi * 15
phase_mod = np.sin(rho * const) * (np.sqrt(2) / 25)
pupil2.phase += phase_mod
ps = PSF.from_pupil(pupil2, efl=50, Q=1)
ps.plot2d(xlim=200, power=1/3)
[3]:
(<Figure size 432x288 with 2 Axes>,
<AxesSubplot:xlabel='Image Plane X [$\\mathrm{\\mu m}$]', ylabel='Image Plane Y [$\\mathrm{\\mu m}$]'>)
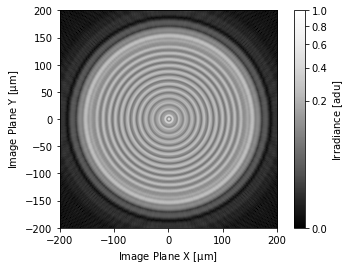
The ripples print through into the in-focus image. What if the image was in focus?
[4]:
pupil3 = NollZernike(samples=384, dia=25) # same parameters as before, but no error
pupil3.phase += phase_mod
pupil3.plot2d(clim=0.25) # +/- 138 nm
plt.title('Wavefront with ripple error')
ps = PSF.from_pupil(pupil3, efl=50, Q=3) # Q=2 now, we need high resolution
ps.plot2d(xlim=20, power=1/4)
[4]:
(<Figure size 432x288 with 2 Axes>,
<AxesSubplot:xlabel='Image Plane X [$\\mathrm{\\mu m}$]', ylabel='Image Plane Y [$\\mathrm{\\mu m}$]'>)
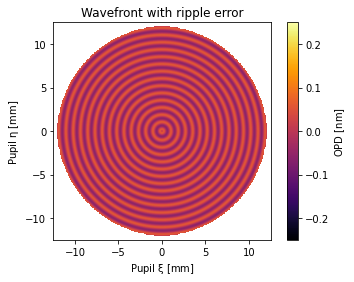
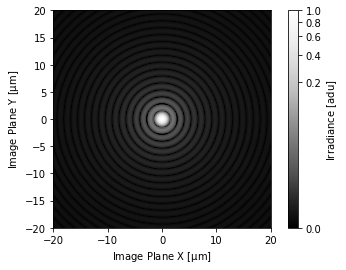
This looks just like an Airy disk; the ripples have low RMS amplitude, so they do little damage to the in-focus image.
What if the ripples were more localized, occuring in just one band within the clear aperture?
[5]:
def gauss_r(r, center, sigma=0.1, amplitude=1):
numerator = (r-center) ** 2
denominator = 2 * sigma ** 2
return amplitude * np.exp(-numerator / denominator)
phase_mod2 = phase_mod * gauss_r(rho, center=0.66, sigma=0.02)
phase_mod_vis = phase_mod2.copy()
phase_mod_vis[pupil.transmission == 0] = np.nan
plt.imshow(phase_mod_vis, cmap='inferno')
pupil4 = pupil.copy()
pupil4.phase += phase_mod2
ps = PSF.from_pupil(pupil4, efl=50, Q=1.25) # Q=2 now, we need high resolution
ps.plot2d(xlim=200, power=1/3)
[5]:
(<Figure size 432x288 with 2 Axes>,
<AxesSubplot:xlabel='Image Plane X [$\\mathrm{\\mu m}$]', ylabel='Image Plane Y [$\\mathrm{\\mu m}$]'>)
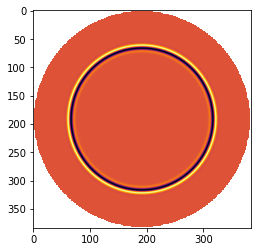
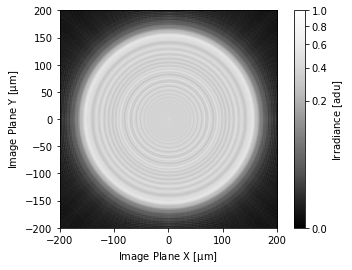
Now there is an inner ring in addition to the Fresnel rings. The formation of this is related to the angular spectrum of the aperture. An ensemble of rays from the perturbed annulus of the aperture propagate at a different angle to the rest; the interference begins at the radius they appear at within the clear aperture, crosses through the center at focus where they overlap with the natural interference from the support of the aperture, then dissipate to an infinite distance as the observation plane moves further behind focus.